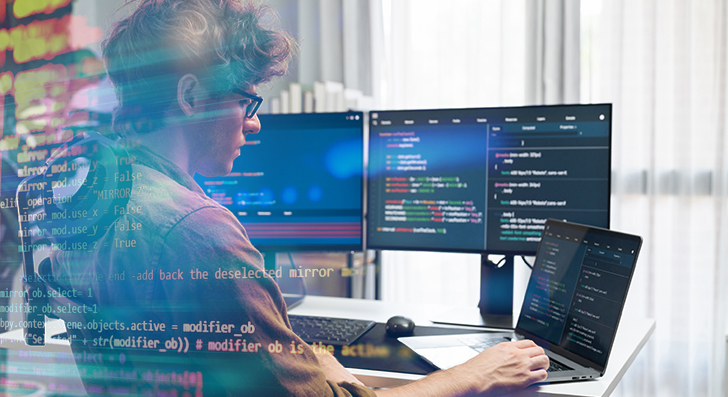
Scalability implies your software can cope with progress—a lot more users, extra facts, plus much more website traffic—devoid of breaking. Like a developer, building with scalability in your mind saves time and anxiety later. Below’s a clear and practical tutorial that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not something you bolt on later on—it should be aspect of one's system from the beginning. Lots of programs are unsuccessful every time they expand speedy due to the fact the original layout can’t handle the extra load. To be a developer, you should Feel early regarding how your system will behave under pressure.
Get started by developing your architecture to generally be flexible. Prevent monolithic codebases exactly where almost everything is tightly connected. As a substitute, use modular style or microservices. These designs crack your application into smaller sized, impartial pieces. Every module or provider can scale on its own with no influencing the whole system.
Also, consider your databases from working day one. Will it want to handle a million end users or merely 100? Choose the proper form—relational or NoSQL—based on how your info will increase. Strategy for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Another essential position is to stay away from hardcoding assumptions. Don’t generate code that only functions below present-day conditions. Take into consideration what would come about When your consumer foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use design and style styles that aid scaling, like information queues or party-pushed devices. These assistance your application cope with additional requests devoid of getting overloaded.
When you build with scalability in your mind, you are not just planning for achievement—you're reducing future problems. A very well-prepared procedure is less complicated to keep up, adapt, and develop. It’s much better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the correct databases is often a essential Portion of developing scalable purposes. Not all databases are designed precisely the same, and using the Completely wrong you can sluggish you down or perhaps cause failures as your application grows.
Commence by understanding your facts. Could it be highly structured, like rows in a very table? If Certainly, a relational databases like PostgreSQL or MySQL is an efficient match. These are solid with relationships, transactions, and regularity. They also aid scaling tactics like read replicas, indexing, and partitioning to manage far more visitors and facts.
Should your details is much more adaptable—like user action logs, item catalogs, or paperwork—think about a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured details and may scale horizontally additional effortlessly.
Also, look at your study and publish styles. Will you be performing a great deal of reads with much less writes? Use caching and read replicas. Have you been managing a heavy compose load? Check into databases that can manage higher compose throughput, or maybe event-primarily based knowledge storage units like Apache Kafka (for temporary information streams).
It’s also sensible to Assume in advance. You might not will need Highly developed scaling features now, but selecting a database that supports them implies you gained’t will need to modify afterwards.
Use indexing to hurry up queries. Avoid unneeded joins. Normalize or denormalize your facts based upon your obtain styles. And always monitor database overall performance as you expand.
In a nutshell, the correct databases will depend on your application’s framework, pace demands, And just how you assume it to increase. Just take time to choose properly—it’ll conserve lots of difficulties later on.
Optimize Code and Queries
Quick code is key to scalability. As your application grows, just about every smaller hold off adds up. Poorly written code or unoptimized queries can decelerate performance and overload your system. That’s why it’s important to Establish successful logic from the start.
Begin by writing clean, very simple code. Prevent repeating logic and remove something needless. Don’t choose the most advanced Resolution if a simple a person will work. Maintain your capabilities small, targeted, and straightforward to check. Use profiling resources to locate bottlenecks—places wherever your code will take too very long to run or takes advantage of excessive memory.
Subsequent, evaluate your database queries. These often sluggish items down more than the code by itself. Make certain Just about every query only asks for the info you actually need to have. Steer clear of Pick out *, which fetches every thing, and in its place pick particular fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Specifically throughout large tables.
In case you see the identical details becoming asked for many times, use caching. Retail outlet the results temporarily working with applications like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database operations any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and will make your app a lot more productive.
Make sure to exam with large datasets. Code and queries that perform wonderful with a hundred documents might crash once they have to deal with 1 million.
To put it briefly, scalable applications are fast apps. Keep your code tight, your queries lean, and use caching when required. These measures support your software keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it has to handle more customers and much more site visitors. If every little thing goes by way of one particular server, it is going to speedily turn into a bottleneck. That’s the place load balancing and caching are available in. These two tools support maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of one particular server undertaking each of the perform, the load balancer routes customers to different servers based on availability. This means no one server will get overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing information quickly so it could be reused swiftly. When consumers request a similar data once more—like an item website page or perhaps a profile—you don’t really need to fetch it through the database anytime. It is possible to serve it through the cache.
There are two popular different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapid access.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents close to the consumer.
Caching reduces database load, increases speed, and tends to make your application much more successful.
Use caching for things that don’t adjust often. And constantly make sure your cache is up to date when details does alter.
Briefly, load balancing and caching are easy but strong tools. Collectively, they help your app cope with more end users, continue to be fast, and Recuperate from challenges. If you plan to expand, you require both.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need equipment that allow your app develop simply. That’s where by cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and expert services as you would like them. You don’t have to get components or guess long run potential. When targeted visitors increases, you are able to include much more sources with only a few clicks or instantly making use of automobile-scaling. When site visitors drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety instruments. You could deal with making your application as an alternative to controlling infrastructure.
Containers are Yet another important tool. A container offers your application and almost everything it should run—code, libraries, settings—into a person device. This makes it simple to move your application involving environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application makes use of numerous containers, applications like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it quickly.
Containers also ensure it is easy to different areas of your application into companies. You are able to update or scale pieces independently, that's great for effectiveness and reliability.
To put it briefly, making use of cloud and container tools signifies you can scale rapid, deploy effortlessly, and Get well rapidly when issues transpire. If you would like your application to grow with no limits, start off using these equipment early. They help you save time, decrease possibility, and help you keep centered on building, not repairing.
Watch Every thing
In case you don’t observe your application, you received’t know when things go Improper. Checking allows you see how your app is doing, location issues early, and make far better selections as your application grows. It’s a vital A part of constructing scalable devices.
Start by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These show you how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—check your app way too. more info Control just how long it will require for people to load internet pages, how frequently faults materialize, and where by they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital issues. As an example, Should your response time goes over a limit or a company goes down, you'll want to get notified straight away. This allows you deal with troubles rapidly, usually just before customers even notice.
Checking is likewise valuable once you make modifications. If you deploy a completely new element and see a spike in problems or slowdowns, you are able to roll it again in advance of it brings about genuine damage.
As your application grows, site visitors and data maximize. With no monitoring, you’ll pass up signs of trouble until eventually it’s also late. But with the right instruments in place, you continue to be in control.
To put it briefly, monitoring helps you maintain your app reputable and scalable. It’s not just about recognizing failures—it’s about comprehension your method and making certain it works properly, even under pressure.
Ultimate Thoughts
Scalability isn’t just for significant organizations. Even compact apps have to have a powerful Basis. By designing meticulously, optimizing wisely, and using the suitable resources, you may Develop applications that mature easily devoid of breaking under pressure. Commence smaller, Consider significant, and Develop clever.